Find length of the largest subarray with sum 0
- ishitajuneja01
- Mar 22, 2023
- 4 min read
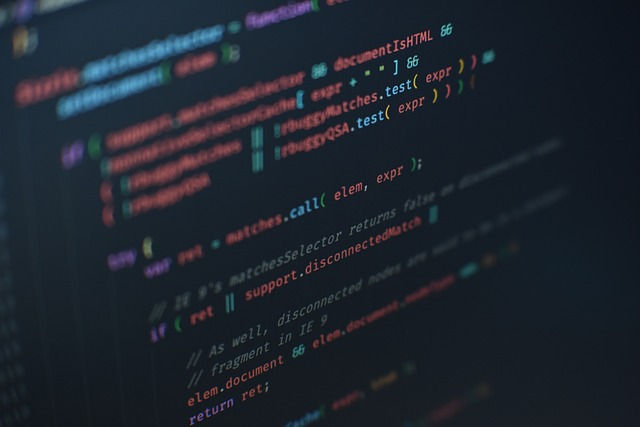
If you're an aspiring programmer, you would know that array data structures are explored in almost all programming languages.
Additionally, the Subarrays concept is one of its prominent core, which is frequently asked in interviews.
A Subarray is usually referred to as an array within an array because it is utilized in a program to store data that may be used for additional repetitive purposes.
Subarrays are also available in two sizes: small and large.
Though, in terms of large subarrays, how will you determine the length of the largest subarray with 0 sum?
Well, there are two basic methods: brute force and HashTable.
We all would know about Brute force since it is used commonly to solve problems like two sum, coin change and such. However the HashTable is another unique method which we will learn thoroughly about in the following blog.
Let’s dive in!
What is the largest subarray with 0 sum problem all about?
You will be provided with an array of size which will include both positive and negative digits. From the given array, you will be asked to find the largest subarray with sum 0.
To understand this problem, we should first understand the subarray problem as a whole. Previously we saw how the subarray is used to store elements in the original array’s form. Now it is evident that subarray and array’s nature are literally the same.
Here’s an example to understand a subarray.
Input: arr [1,2,3,4]
The subarrays of the input array would be: [1],[1,2],[1,2,3],[1,2,3,4],[2],[2,3],[2,3,4],[3],[3,4],[4]
Now we understand how subarrays are formed. But when it comes to largest subarray with sum 0, we can understand it by,
Input:
arr [4,-3,-6,5,1,6,8]
Output: 4
Explanation: The size of the array is 7. Now we have to find the subarrays from ith index to jth index (0<=i<7 and i<=j<7).
The subarrays with 0 sum which we would get are:
Length 3 arrays are [−6,5,1] and Length 4 arrays are [4,−3,−6,5].
When we compare these two sets, the largest subarray with 0 sum is 4.
Hopefully, you now understand the subarray concept clearly. Now let us check out the methods through which we can approach this sum.
Methods to find the largest subarray with 0 sum
The best methods to find the solution to this problem would be brute force and hashtable. Under brute force method, we will have two sub-approaches, such as
Brute Force: Three Nested Loop Approach
In this method, once we find all the subarrays, we will find and check each subarray’s sum. Then, we will save the largest subarray’s length and return the answer.
Steps:
Initiate and declare the variable function max_length = 0.
Once you do this, it will store the length of the largest subarray that has sum 0.
Now, start traveling through an array from its beginning point.
For the array’s i indexes, you should initiate a nested loop with j variable. The j variable must range from i index to the array’s size.
Now initiate, variable cursun=0.
This will store the subarray’s sum which starts from the ith index that also ends in the jth index. To find the sum, you should run a loop.
Analyze and see if the cursum's value is equal to 0. If the present subarray’s length seems to be greater than the length that is saved in max_length, change it with the length of the present subarray.
Finally, return max_length.
Brute Force: Two Nested Loops
In this method, we will use two nested loops to compute the subarrays sum. In two loops, we will fix the outer loop at the subarray’s starting index and the inner loop will be set at the subarray’s ending index.
While running the second loop, we will figure out the sum and save its length.
Steps:
First initiate the max_length variable which will save the largest subarray with sum 0 length.
Now traverse the array from the start index while initiating the cur_sum variable which will save the subarray’s sum which starts at with index.
Now traverse the array from the preceding element of the present index until it reaches the array’s end.
You should figure out whether cur_sum is equal to 0. Check if the firstly initiated max_length is lesser than the present subarray’s length. If so, change the max_lenght’s value to the present subarray’s length value.
Return max_length.
Hash Table
In the Hash Table method, we will use hashing structure to iterate over the given array and find the sum of the characters from the array’s starting to the end. We will then check if the present sum is also available in the Hash Map which we will initiate.
Steps:
First, initiate the max_length variable like how its done in the above approaches. The max length value must be equal to 0.
Then initiate cur_sum by also keeping its value as 0.
Initiate an empty hashmap to save all the previous sum-index values which we will consider as key-value pairs.
Now start the iteration on the input array.
In every index, keep adding the cur_sum to the sum. The formula would be cur_sum + array [i].
For each index, keep checking whether in the hashmap we have the current sum.
If the current sum is found in the hash map, then change the max_length to the maximum max_length and also the difference between current index and hash-map index.
Once you change the values as we said previously, you should return the maximum length for the output desired.
Final Thoughts
Alright that’s a wrap!
Subarrays are really a great concept to learn about because they are mostly asked in interviews. We hope our step by step guide to the methods have helped you solve complex questions.
If there’s any other doubt regarding sums like beautiful numbers, coin change, house robbers and much more!
Head to our website because we have some of the finest free resources to learn from.
Comments