How to add one array to another array in Python?
- ishitajuneja01
- Feb 17, 2023
- 4 min read
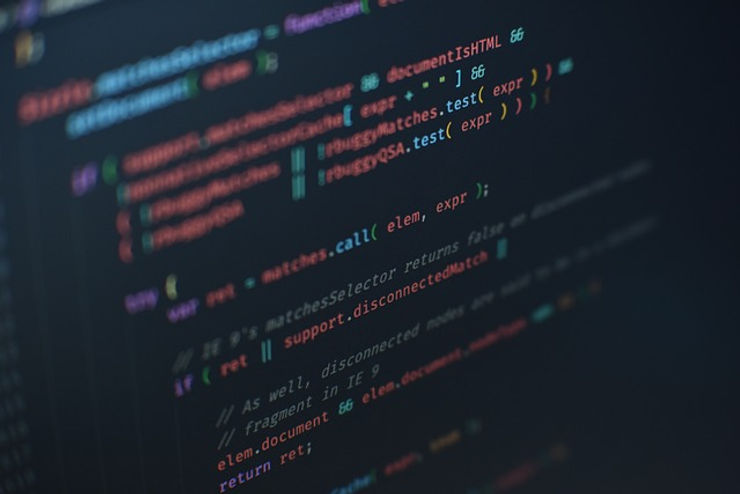
Whether you are preparing hard for a coding interview or want to ace coding like a pro or just entering the coding world; you can’t escape the concept of array.
Well reasons can be multiple, but the importance of arrays can’t be denied!
Arrays are touted as the components of data structures that enable you to store and work with vast amounts of data.
Though, while dealing with arrays, you need to do various implementations.
Although multiple implementations can be performed, one of the key concepts that is included in the array and is often asked from the aspirants is sum of two arrays in python.
The most important question can be, how can you add one array to another array?
Python is a versatile language that offers a number of methods to achieve desired result, including concatenating two arrays, stacking them on top of one another, and simply adding their items.
Read this blog to gain knowledge momentum on this topic!
What is an Array?
An array is defined as a data structure that holds a group of objects, typically of the same type.
Its elements are kept in consecutive memory regions and can be retrieved either using their index number or their position within the array.
In programming, arrays are frequently used to store groups of data that can be handled consistently and logically. A list of integers, characters, or other data kinds can be stored in an array and retrieved and modified as required.
Steps to add one array to another array in Python
To find sum of two array in python you need to follow certain steps which are discussed as below:
First, establish two arrays: First generate two arrays that you wish to combine before you can add them. List data types in Python can be used to generate arrays.
For example:
array1 = [1, 2, 5, 4]
array2 = [9, 6, 7, 8]
Concatenate the arrays: Python's "+" operator can be used to combine two arrays into one large array.
Take another example:
result = array1 + array2
Print the outcome: You can utilise the print function in order to view the outcome of the addition.
For example: print(result)
Different ways to add one array to another array in Python
There are various techniques which help determine the sum of two arrays in python. Learn these techniques and apply them in practicality:
Concatenation using the "+" operator
The "+" operator is used for concatenation, which is the simplest way to combine two arrays in Python.
This operator is useful to combine two arrays within a single array. Each element from both arrays will be present in the final array in the same order as in the original arrays.
Let’s understand with an example.
The "+" operator in Python can be used to combine two arrays. By doing this, the two arrays will be combined into one array.
For example:
array1 = [1, 2, 3, 7, 5]
array2 = [6, 4, 8, 9, 10]
result_array = array1 + array2
print(result_array)
Result: [1, 2, 3, 6, 5, 4, 7, 8, 9, 10]
extend method
One can combine two arrays by using the extend method. The extend method effectively joins two arrays together by adding the elements of a single array to the end of the other array.
This is also an effective way to determine a happy string within an array.
For example:
array1 = [1, 2, 9, 4, 5]
array2 = [6, 7, 8, 3, 10]
array1.extend(array2)
print(array1)
Result: [1, 7, 3, 4, 5, 6, 2, 8, 9, 10]
Numpy library
By using the Numpy library, you can find the sum of two arrays in python. Numerous functions including element-wise addition, concatenating arrays, and other operations, are available in Numpy for working with arrays.
To combine arrays, Concatenate and append are two functions used in the numpy library.
For instance:
import numpy as np
array1 = np.array([1, 2, 3, 6, 5])
array2 = np.array([4, 7, 8, 9, 10])
result_array = np.concatenate((array1, array2))
print(result_array)
Result: [ 1 2 8 4 5 6 7 3 9 10]
itertools.chain method
A single, iterable object can be created by combining the components of two or more arrays using the itertools.chain method. The elements of the merged arrays can then all be accessed using this iterable object.
For example:
import itertools
array1 = [1, 10, 3, 4, 5]
array2 = [6, 7, 8, 9, 2]
result_array = list(itertools.chain(array1, array2))
print(result_array)
Result: [9, 2, 3, 4, 5, 6, 7, 8, 1, 10]
List comprehensions
Two arrays can be added using list comprehensions. List comprehensions offer a succinct and effective method to carry out actions on lists and arrays, such as fusing two arrays into one array.
For example:
array1 = [1, 2, 3, 4, 5]
array2 = [6, 7, 8, 9, 10]
result_array = [x for sublist in [array1, array2] for x in sublist]
print(result_array)
Result: [1, 2, 3, 4, 5, 6]
The optimal strategy for adding the two arrays will vary depending on the particular needs of your project because each of these approaches have their own pros and cons.
Understanding a Problem Statement
Combining two arrays into a single array by combining all the elements from both arrays is the goal of the problem statement "How to find sum of two arrays in python."
The issue statement may provide restrictions on the components that must appear in the result array in a specific order, the type of the input arrays (such as list or numpy arrays), and the intended output structure (like list, numpy array, etc.).
Depending on the exact needs of the issue statement, the solution to the problem entails usage of one of the techniques to combine the two arrays.
You can apply the techniques when instead of integers, there are alphabets in arrays just as in happy string.
Here is an illustration showing how to use Python's "+" operator to combine two arrays:
array1 = [1, 2, 3, 4, 5]
array2 = [6, 7, 8, 9, 10]
result_array = array1 + array2
print(result_array)
Result: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In this illustration, there are two lists of integers called array1 and array2. These two lists are combined into one list, result array, which includes every element from both arrays using the "+" operator.
Conclusion
Equip yourself with the knowledge on how to find the sum of two arrays in python with the help of this blog post.
Further, you can enhance your learning on other crucial coding interview concepts like happy strings.
Keep Learning!
Kommentare