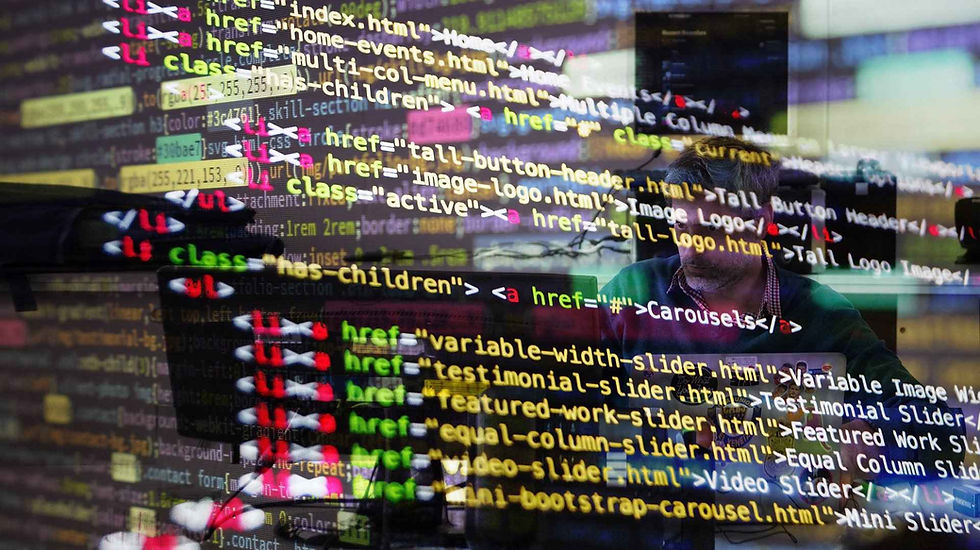
Are you trying to find a missing or repeating number in your code but have been unable to do so?
Are you wondering if there is an easier, more efficient way to solve this problem than the trial-and-error method?
A missing number in an integer array is a number that should be there given the range of values in the array but is not present and any number that occurs more than once within the array is considered to be repeating.
In programming, finding these values are important, so methods like sorting, hashmap and xor operation are being used for this.
Till the end of this blog, we'll explore methods for finding and fixing any missing or repeating numbers that may be causing issues with your program.
We'll discuss the steps that you should follow in each method in case of these problems, and provide examples of how it all works together so that you can quickly identify and resolve these errors yourself. Read on to learn more!
Methods to find numbers that repeat and missing number array
In programming, there are numerous ways to locate the missing number in a series or list of numbers.
Among the most popular methods are:
Method 1: Using Sorting Algorithm
A sorting algorithm in computer science is a method for organising the items on a list. The most often used ordering systems are lexicographical and numerical, both in ascending and decreasing order. The effectiveness of other algorithms (like searching and merge techniques) that need data input to be in sorted lists must be maximised, hence efficient sorting is crucial.
Additionally, canonicalizing data and creating output that is intelligible by humans also benefit from sorting.
Any sorting algorithm's output must formally meet the following two requirements:
The output follows the specified order and is in monotonic order, meaning that no element is smaller or greater than the one before it.
The result is a permutation of the input, which reorders the elements while keeping the original ones.
You can use the steps below to use a sorting algorithm to identify the missing and recurring number:
The input array should first be copied, then sorted in ascending order.
Compare each entry with the one before it as you go over the sorted array. If there is a discrepancy between the current node and the preceding element that is greater than 1, the previous element plus one is added to get the missing number.
If there is a difference of one between the input array and the one before it, the array iteration will continue.
When there is no difference between the present element and the one before it, the repeating number serves as the input array.
Due to the sorting phase, this method has a temporal complexity of O(n * log(n)), making it appropriate for smaller to medium-sized arrays. You might want to take into account adopting a different strategy, such as employing a hash table or a set of numbers, if you require a speedier solution for larger arrays.
Method 2: Using a hash map
To find the numbers that repeat and missing number array using a hash map, you can follow these steps:
To store the total of each integer in the array, create a hash map.
Update the hash map's count of each number as you iterate through the array.
Repeat the array iteration process and count every number using the hash map. If there are more than one, it indicates that the number is recurring. A missing number is indicated with a count of 0.
Bring back the erroneous and repeated numbers.
Method 3: Using XOR Operation
To find the numbers that repeat and missing number array using a XOR Operation, you can follow these steps:
XOR each and every element in the array. Let xor arr be the outcome.
XOR every digit from 1 to n. Let xor n be the outcome.
By XORing xor arr and xor n, the missing and repetitive numbers can be found. Let xor man be the outcome.
You can employ the following expression to determine which set bit in xor mn is rightmost: xor mn & (xor mn - 1); rightmost set bit;
Separate the array's numbers into 2 groups: one group for those whose rightmost set bit is set and another group for those whose rightmost set bit is unset.
Each group's numbers are separately XORed. Let xor set and xor unset represent the outcomes, respectively.
By XORing xor set and xor unset, the missing and repetitive digits can be found.
def find_missing_and_repeating(arr, n):
xor_arr = 0
xor_n = 0
for i in range(n):
xor_arr ^= arr[i]
xor_n ^= i+1
xor_mn = xor_arr ^ xor_n
rightmost_set_bit = xor_mn & ~(xor_mn - 1)
xor_set = 0
xor_unset = 0
for i in range(n):
if arr[i] & rightmost_set_bit:
xor_set ^= arr[i]
else:
xor_unset ^= arr[i]
for i in range(1, n+1):
if i & rightmost_set_bit:
xor_set ^= i
else:
xor_unset ^= i
for i in range(n):
if arr[i] == xor_set:
return (xor_set, xor_unset)
return (xor_unset, xor_set)
The time complexity of this approach is O(n) and the space complexity is O. (1).
It should be noted that this method assumes the array contains just one recurring and one missing integer. This strategy won't work if there are multiples of either.
How to find the numbers that repeat and missing number array?
You can use the steps below to determine the numbers that repeat and missing number array in a palindromic subsequence:
To keep track of how many numbers are in the palindromic subsequence:, use a list or array.
Increase the count of each value in the list or array as you iterate over the subsequence.
Check which number in the list or array has a count of 0 to locate the missing value.
Look for the number that appears more than once in the list or array to identify the repeating number.
Here is an illustration of how you could do this in Python:
def find_missing_and_repeating(subsequence):
count = [0] * len(subsequence)
for number in subsequence:
count[number] += 1
missing = -1
repeating = -1
for i, c in enumerate(count):
if c == 0:
missing = i
elif c > 1:
repeating = i
return missing, repeating
The missing and recurring numbers are returned as a tuple by this function, which accepts a palindromic subsequence as input. The for loop repeats throughout the subsequence to refill the count array, which is used to hold the count of every number in the subsequence.
The missing value is then located by searching for the value with a total count of 0, while the recurring number is located by searching for the value with a count higher than 1.
Conclusion
By understanding and utilising these array search methods, you'll be able to streamline your code and make it more efficient. Rather than wasting time poring over arrays of information manually, you can quickly locate the missing or repeating numbers using these simple techniques. In no time at all, you'll be coding like a pro!
Comments