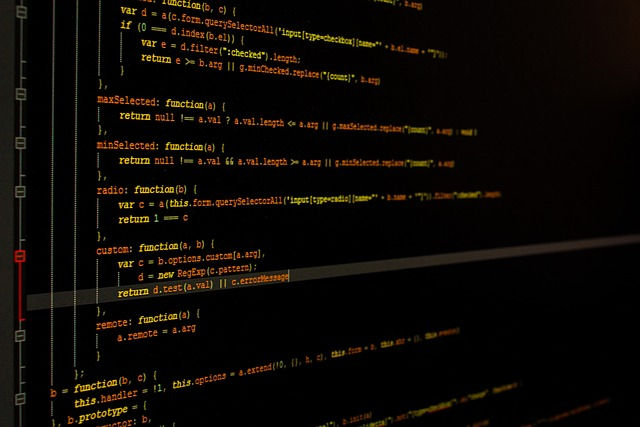
Programming languages that are supported and based on Object-Oriented Programming such as Java have the highest ratings globally in terms of providing faster and time efficient performance.
From Infosys to Wipro, nearly all the prominent tech brands are searching for pro level Java developers. There are huge vacancies all over the world in different positions for people who are efficient in Java.
So, would you consider yourself as efficient in Java? Well, learning Java Development would not be complete unless you learn all of its important concepts.
Evidently, this blog caters towards problem solving one such important concept of Arrays in Java i.e finding the second largest number in an Array.
What do you mean by the second largest number in an Array?
In the context of Data Structures and Algorithms, the arrays can be defined as a sequence or series of data that is arranged in a lexical order.
An array essentially consists of a collection of items or elements that reflect similar characteristics. These elements can either be numbers or letters that are usually arranged in ascending or descending orders.
For full disclosure, the arrays are usually not arranged in a sorted order.
This is why, before implementing any sort of logic or function in an array, it is important to make sure that we prioritize the sorting the array in a proper order.
That said, when we are given an array of integers, the second largest number in the lot will essentially be the second largest integer of the array.
Now, in order to figure out the second largest element in an array, we can effectively apply the Brute force Algorithm and implement the Map Data Structure.
We have elaborated further on this concept in the next section of our blog.
What methods can be used for finding second largest elements in an array?
We have already mentioned two different approaches for finding the second largest element in array for a given series of integers.
But, that is certainly not all. We also have a few different approaches that are based on traversing the array and increasing the efficiency for time and space.
Let's find out how these methods would be implemented for the data structure.
Method 1: Using the Naive Approach or Brute Force Algorithm
Have you come to notice that the Brute Force Algorithm is essentially used in every other programming problem?
From merging two sorted arrays to finding the longest common prefix in a string, the Brute Force Algorithm is present everywhere!
Well, in this context, the general idea is to sort the given array in a descending order of elements and finally print the second element that is found to be not equal to the largest element of the array.
Method 2: Implementing the Efficient Approach
This approach focuses on traversing the array twice. While we are on the frost traversal we will try to locate the maximum element value.
On the other hand, when the second traversal resumes, we shall be finding the second largest element in the array.
Method 3: Initialising the Map Data Structure
This approach in DSA is also commonly used in the longest common prefix problems for a string.
By initialising the map data structure, we will essentially be creating a map of a larger size than the given input data and, by iterating on this map, we will be able to figure out the size of the second largest element in the array.
How to apply the algorithms for finding the second largest number in an Array?
Now, this section of the blog will further discuss the process of implementing the methods that we have previously mentioned in detail.
Method 1: Implementing the Naive Approach or Brute Force Algorithm
Check out how the implementation for the Brute Force Algorithm would work for finding the second largest element in array.
Firstly, begin with sorting the given array in a descending order of characters.
Next up, you will have to check for the value of the largest element in the newly sorted array.
Finally, once the largest element is found, it will be easier to look for the second largest element by iterating the Brute Force Algorithm once again in the program.
Return the value of the second largest element found in the array.
Time Complexity for this approach:
O(n log n)
Method 2: Implementing the Efficient Approach
Here's how the algorithm for the array traversal method will be implemented:
Begin the first traversal of the array by invoking the traversal function in the program.
Find the value of the largest element in the first traversal itself.
Next up, initiate a second traversal where our motive will be to find the second largest element from the remaining elements of the array.
Time Complexity for this approach:
O(n)
Method 3: Initialising the Map Data Structure
Finally, here's how the algorithm for initialising the map data structure should be executed:
Start with initialising a map data structure.
Next up, you may start storing the values of the elements in the map data structure. Always remember to manage the data structure in an increasing order.
If the size of the map is greater than 1 you can rest assured that the second largest element does exist in the map.
Finally, you can return the second largest element.
Time Complexity for this approach:
O(n)
Final Thoughts
It is certainly evident that the interviewers of technical interviews have more affinity toward some programming languages than others.
Java definitely tops the list for being one of the popular choices amongst both the interviewers as well as the applicants.
Why so?
Well, efficiency in executing programming concepts is definitely one of the biggest factors that has contributed to the popularity of Java.
From the longest common prefix problems to string compression, there are absolutely no problems that cannot be solved by Java within a reduced time complexity!
Comments